Chrome Debugging Tips
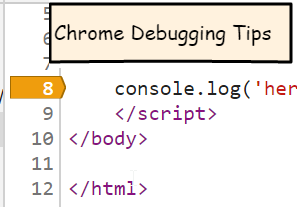
This post covers some useful tips for debugging JavaScript in Chrome.
Tip1 – Output objects in console.log The console.log statement is used inside of source code, usually as part of development to help debug a section of code such as console.log(‘hit this point’); or console.log(‘my variable is ‘ + x); One useful trick when working with the console.log statement is that you can output a complete object by using the %o (that’s the letter o). Here is an example.
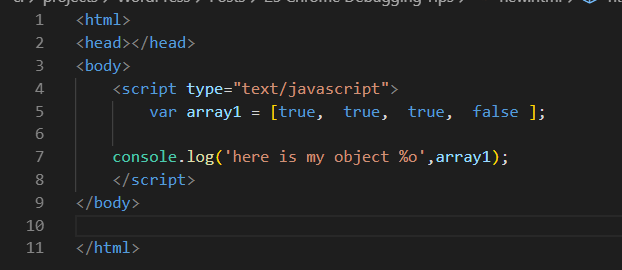
What this gives us in the browser is not just the text, but the whole object structure. So you can click the dropdown to navigate through the entire object.
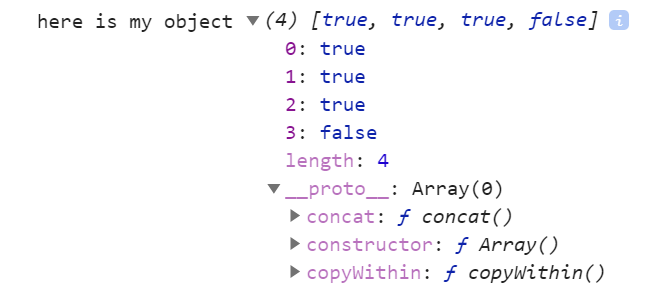
Tip 2 – Conditional breakpoints. Most people who have worked with JavaScript in Chrome are familiar with setting breakpoints. In the sources tab, click the gutter on the left-hand side of the code you would like to add a breakpoint to. However, did you know you can also quickly add conditional breakpoints to be more selective about when your breakpoint fires?
To turn a regular breakpoint into a conditional breakpoint, right click the breakpoint and then select the ‘edit breakpoint’ option.
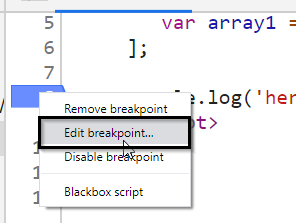
A dialog will appear that lets you type in a conditional statement.

After adding a conditional statement you will notice that the breakpoint has updated from blue to yellow. This breakpoint will only apply when the condition statement is met (evaluates to true).
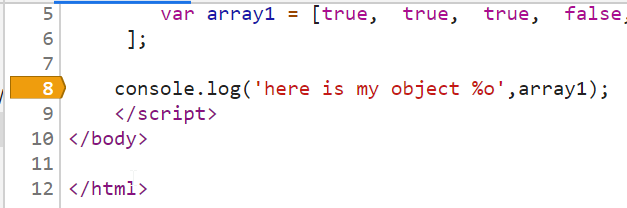
Tip 3 – Blackboxing scripts. It is very common to use additional JavaScript libraries or even full frameworks as part of web development. For example here I’m showing an example of the jQuery library. If I’m debugging and I click ‘Step Into’ on this line I end up inside the source code of jQuery.
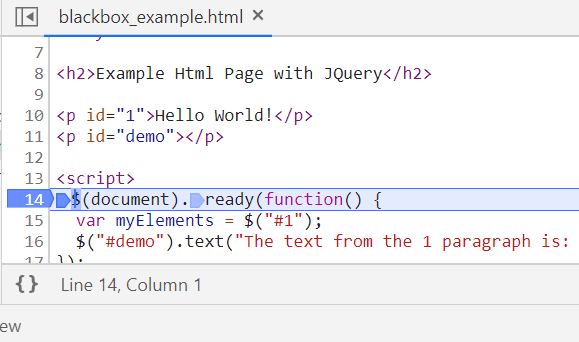
Generally you’re only concerned with troubleshooting your own code, so when this happens you can right click within the library or source code file you want to ignore and click the ‘Blackbox script’ option.
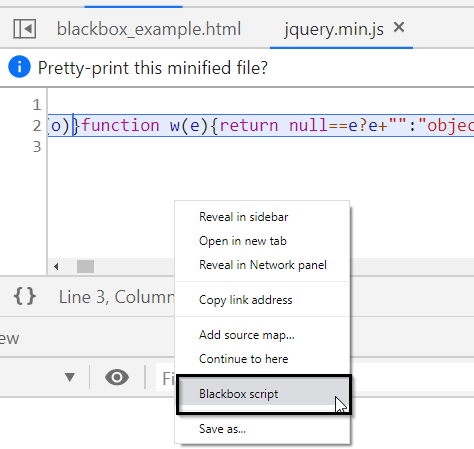
After the blackbox has been applied any ‘Step Into’ actions you apply will execute the code without stopping the debugger. This allows you to focus just on your own code.