Vue.js conditional display v-if and v-show
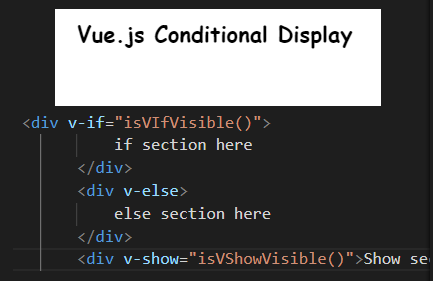
In working with the Vue.js JavaScript framework, one of the first topics you learn is conditional display – displaying or hiding an element. Two ways to do this are the v-if directive and the v-show directive.
Here is an example of the v-if directive:
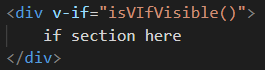
It’s pretty straight forward, the statement inside the quotes needs to evaluate to a true or false aka boolean value. True and the element will display, false and the element will not display. A best practice with the v-if directive would be to call a method that returns a boolean versus in-lining more complex code.
Along with the v-if directive you can also use the v-else directive as shown here:
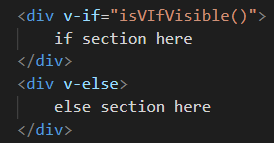
The syntax for v-show is the same as v-if, however v-show does not work with the v-else directive.

Here is the complete code sample for this article that demos both v-if and v-show. To run the sample, simply copy the code into an index.html file and open it in a browser.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
<meta charset="utf-8"><title>Vue.js conditional display example</title> <div id="app"> <button @click="toggleVIfVisible">Toggle v-if</button> <button @click="toggleVShowVisible">Toggle v-show</button> <br> <div v-if="isVIfVisible()"> if section here </div> <div v-else=""> else section here </div> <div v-show="isVShowVisible()">Show section here</div> </div> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script type="text/javascript"> var app = new Vue({ el: '#app', data: { local:{ isVIfVisible:true, isVShowVisible:true } }, methods: { toggleVIfVisible(){ this.$data.local.isVIfVisible = !(this.$data.local.isVIfVisible); }, toggleVShowVisible(){ this.$data.local.isVShowVisible = !(this.$data.local.isVShowVisible); }, isVIfVisible(){ return this.$data.local.isVIfVisible; }, isVShowVisible(){ return this.$data.local.isVShowVisible; } } }) </script> |
The sample provides two buttons you can use to toggle the if and show sections on and off to test out these features.
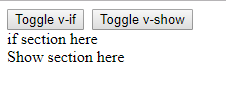
The interesting difference between v-if and v-show is how they actually manipulate the DOM. when v-if evaluates it actually removes the entire element from the dom when the element is not visible and inserts an element in the dom when visible. We can see this with the sample code, click the button to toggle the if statement and take a peek at the dom as you’re testing (F12 in Chrome, Elements tab).
Next lets take a look at what is happening with the v-show directive. When v-show evaluates to false, it does not actually remove the element, it just updates it with a css style display:none, and removes the style when the v-show directive evaluates to true.

The v-show directive should be used in cases where it is likely the display of an element is likely to toggle on and off. This would avoid the overhead of completely rebuilding the element. (especially more complex elements such as components that could have a lot of data or line items). The v-if directive should be used in situations where there is a good chance a given element will not be displayed for a given user. A v-show directive will always build the element, so if it never gets hit, the work to build the complete element only to hide it’s display is wasted compute / bandwidth.
Another way to look at v-show versus v-if would be initial load versus loading when a condition changes later. All elements as a v-show would result in higher initial load times and a fast response as elements toggled. Having all elements as a v-if directive could make initial load faster, but incur more overhead as elements are clicked or changed.
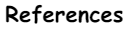