C# Replace Out Parameters with Tuples
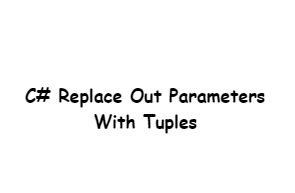
One bit of background, in C# there are actually two different Tuple classes. This post is referring to the System.ValueTuple class which is mutable (changeable). There is also a System.Tuple class which is immutable (read only). See the references link if you are interested in additional details.
Sometimes with C# methods you may set up one or more parameters as ‘out’ parameters. This changes that parameter to a ‘by reference’ type, allowing you to modify the value of the parameter. An example of an out parameter is shown below. In this case we have a method which returns a boolean to indicate success or failure and uses an out parameter to also return a message.
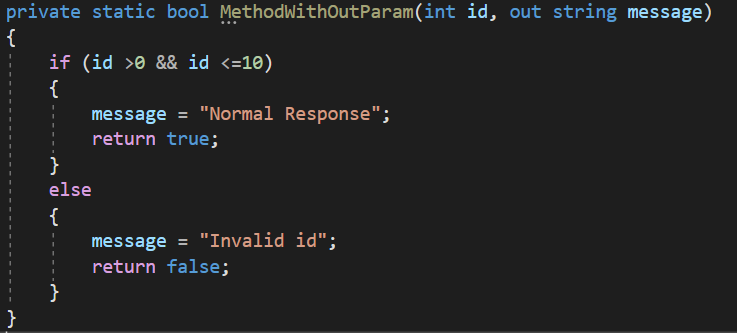
Let’s look at an alternate approach to this problem using a Tuple as a return type.
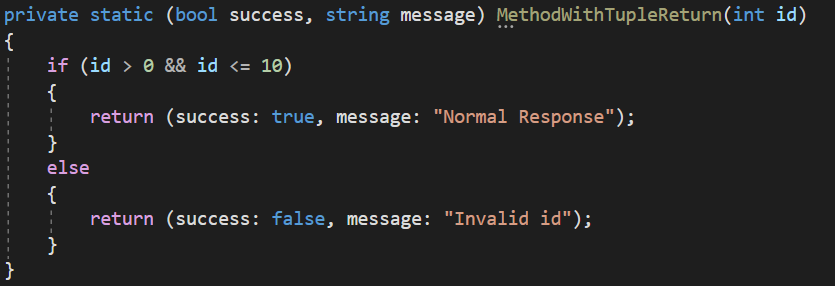
Personally, i think this approach improves code quality a fair bit. We avoid mixing by value and by reference (out) parameters, all of the output is part of the return value.
I would position the use of Tuples for return types like this.
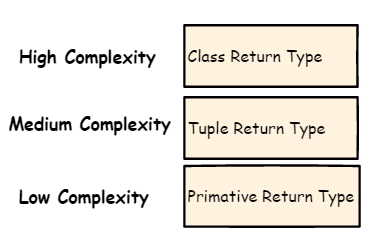
For high complexity return types, use of a full C# class for the return type is appropriate. For low complexity situations with a single return value, a primitive such as a bool or a string will work. I see Tuple return types as a solution for the middle ground. In situations where a class based solution is overkill, but you need to return more than a single value, you should consider a Tuple based return type.
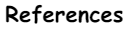
https://docs.microsoft.com/en-us/dotnet/csharp/language-reference/builtin-types/value-tuples