C# Simplify Validation With Guard Clauses
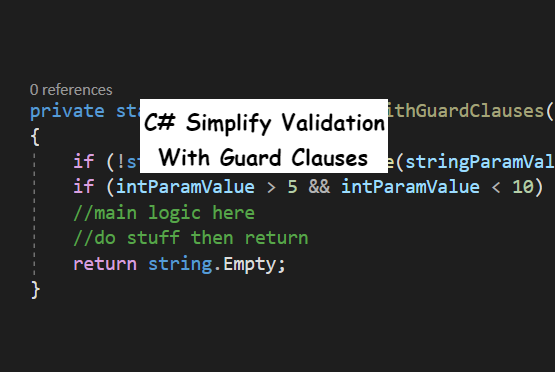
A guard clause is a coding approach for pulling out validation and edge case checks scattered throughout a method and putting them at the start of the method (to validate input parameters) and / or at the end of a method (to validate output after the main processing has taken place in a method). My examples here are in C#, but you can apply this approach to many programming languages. The advantage of this approach is that it can improve code quality and improve the readability and maintainability of your code.
Let’s take a look at a typical method without guard clauses doing some validation, setting a value and then returning a result.
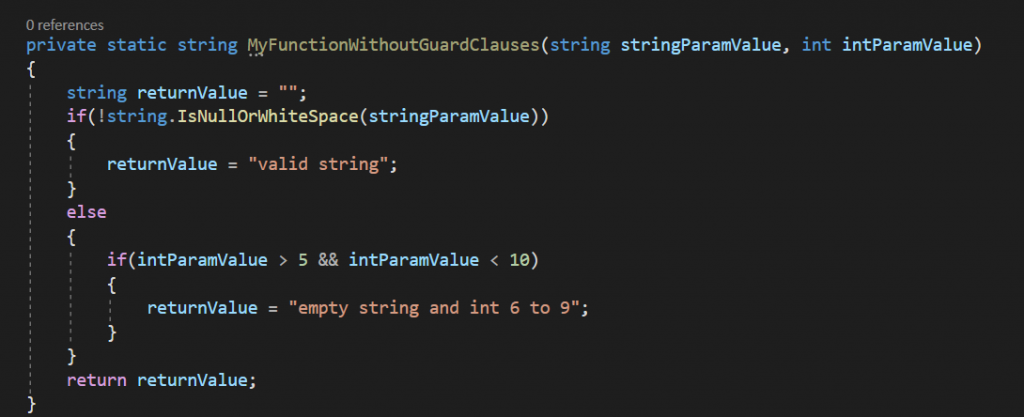
So pretty standard code, let’s look at how this code could be improved by using the guard clause approach.
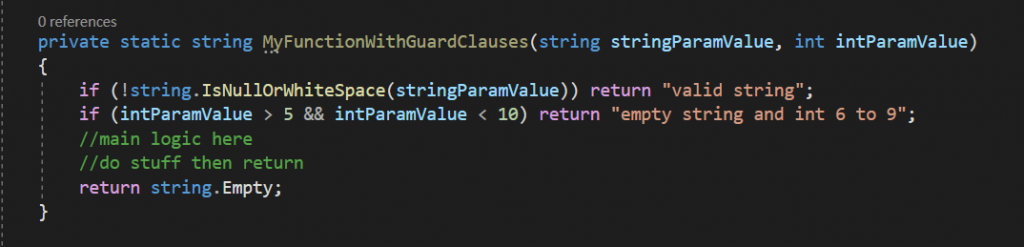
By pulling out all of the validation checks from throughout the method we can remove a number of nested conditionals. This definitely improves the readability of the code and makes the code easier to maintain in the future.
When you start using guard clauses you may start with a code design such as the approach taken above. If you find yourself repeating guard logic frequently you may wish to build your own static ‘Guard’ class as shown.
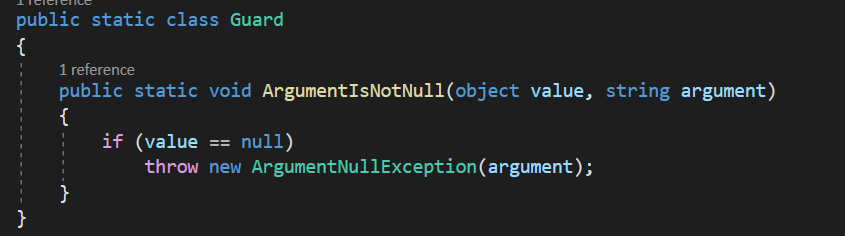
You would then call this guard class like this.

Finally there are also some guard clause libraries that you can utilize that can pull into your project to assist with processing guard clauses.
Additional References: